Software Engineering Fundamentals
Software engineering principles and practices are crucial for successful software development. These notes provide a foundational understanding of software development life cycle models, requirements analysis, and specification, encompassing various methodologies and best practices. The notes cover essential concepts and techniques for building robust, reliable, and maintainable software systems, drawing from widely used resources and academic materials. They serve as a valuable reference for both students and professionals seeking to improve their software engineering skills. The importance of systematic approaches and quality assurance is emphasized throughout. Downloadable PDF versions of these lecture notes are readily available online. Access to these resources can enhance your understanding of software engineering principles.
Introduction to Software Engineering
Software engineering, a systematic approach to software development, differs significantly from mere programming. It involves a disciplined and structured process encompassing planning, design, implementation, testing, and maintenance. These notes delve into fundamental concepts, emphasizing the importance of a well-defined process to create high-quality, reliable, and maintainable software. The field encompasses various methodologies, each with its own strengths and weaknesses, from the classical waterfall model to iterative and agile approaches. Understanding these methodologies is crucial for selecting the most appropriate method for a given project. The notes also highlight the collaborative nature of software engineering, requiring effective teamwork and communication among developers, stakeholders, and clients. Effective software engineering minimizes risks and maximizes efficiency, delivering software that meets user needs and business requirements. Key aspects explored include software requirements analysis, design principles, testing methodologies, and quality assurance techniques. These foundational principles are applicable across diverse software development projects.
Software Development Life Cycle (SDLC) Models
These notes explore various Software Development Life Cycle (SDLC) models, each representing a distinct approach to software development. The Waterfall model, a linear sequential approach, is contrasted with iterative models like the Spiral model, which incorporates risk assessment and iterative development. Incremental models, building software in small, manageable increments, are also discussed, highlighting their adaptability and reduced risk compared to the Waterfall method. Agile methodologies, emphasizing flexibility and collaboration through iterative sprints, are examined, showcasing their effectiveness in dynamic environments. The notes also touch upon the V-model, which emphasizes verification and validation at each stage. Choosing the appropriate SDLC model depends on project size, complexity, and requirements. The notes emphasize the importance of understanding the trade-offs inherent in each model, considering factors such as cost, time, and risk tolerance. Selecting the right model directly impacts the project’s success. Detailed examples and comparisons are included to facilitate understanding and selection of the most suitable SDLC model for specific development scenarios.
Software Requirements Analysis and Specification
This section delves into the critical process of software requirements analysis and specification; Effective requirements gathering is paramount for successful software projects. The notes detail techniques for eliciting requirements from stakeholders, employing methods such as interviews, surveys, and workshops. Emphasis is placed on documenting requirements clearly and unambiguously, using tools like use cases and user stories. The importance of creating a Software Requirements Specification (SRS) document is highlighted, outlining its structure, content, and purpose. The notes explain how to define functional and non-functional requirements, addressing aspects such as performance, security, and usability. Techniques for validating and verifying requirements are discussed, ensuring that the software meets stakeholder needs and expectations. The notes also cover the challenges of managing changing requirements throughout the development lifecycle. The process of prioritizing requirements and resolving conflicts among stakeholder needs is also explored. Effective requirements management is presented as a key factor in avoiding project delays and cost overruns.
Software Design and Architecture
These notes explore software design principles and architectural patterns, crucial for creating robust and scalable systems. Understanding design principles like modularity and abstraction is key to building maintainable software. Architectural styles such as client-server and microservices are examined. The notes emphasize the importance of choosing the right architecture based on project needs.
Software Design Principles
Effective software design hinges on several key principles. Modularity, a cornerstone of good design, involves breaking down complex systems into smaller, manageable modules. Each module should have a well-defined purpose and interface, promoting code reusability and maintainability. Abstraction hides unnecessary details, allowing developers to focus on essential aspects of the system. Information hiding protects internal module details from external access, enhancing robustness and reducing unintended side effects. Coupling refers to the degree of interdependence between modules; low coupling is desirable, as it simplifies maintenance and modification. Cohesion measures the degree to which elements within a module are related; high cohesion indicates a well-focused module with a clear purpose. These principles, when applied effectively, lead to software that is easier to understand, modify, test, and maintain. Adherence to these guidelines significantly improves overall software quality and reduces development risks. Understanding and employing these principles is paramount to creating high-quality, efficient, and maintainable software systems. Careful consideration of these design principles during the development process is essential for long-term success.
Architectural Patterns and Styles
Software architecture encompasses various patterns and styles that guide the structural design of a system. Choosing the right architectural pattern is crucial for achieving scalability, maintainability, and performance. Common patterns include layered architectures, where the system is divided into distinct layers (e.g., presentation, business logic, data access), each with specific responsibilities. Microservices architecture decomposes the system into small, independent services that communicate with each other, enabling independent deployment and scaling. Event-driven architectures utilize events to trigger actions, providing loose coupling and responsiveness. Model-View-Controller (MVC) is a popular pattern for user interfaces, separating concerns into model (data), view (presentation), and controller (user interaction). Each pattern has its strengths and weaknesses, making the choice dependent on specific project requirements and constraints. Understanding these architectural patterns is crucial for designing robust, scalable, and maintainable software systems. The selection process often involves careful consideration of factors like system complexity, performance needs, and future scalability requirements. Effective utilization of these patterns and styles greatly influences the overall success of the software project.
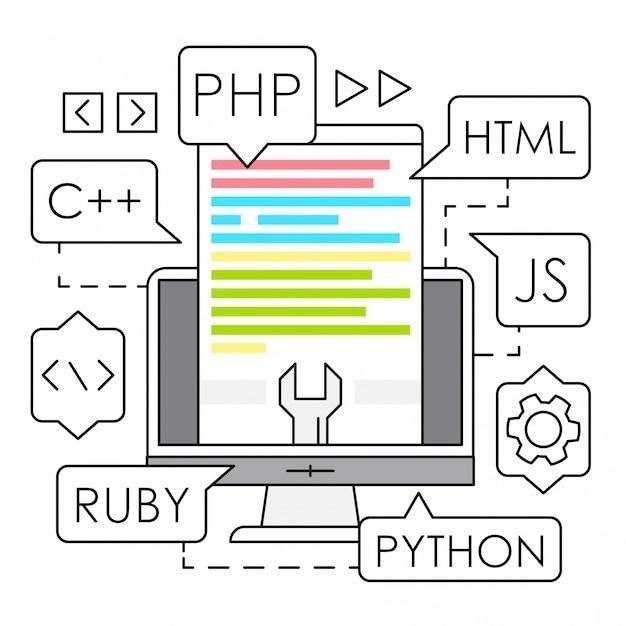
Software Testing and Quality Assurance
Rigorous testing and quality assurance are paramount in software development. These notes detail various methodologies, including unit, integration, and system testing, to ensure software reliability and functionality. They also cover software quality attributes like performance, security, and usability, providing guidance for achieving high-quality software products. Effective testing strategies are crucial for identifying and resolving defects early in the development process.
Software Testing Methodologies
Software testing methodologies are crucial for ensuring software quality. These notes explore various approaches, including black-box testing (functional testing), which focuses on input and output without considering internal program structure, and white-box testing (structural testing), which examines the internal code logic. They delve into unit testing, where individual modules are tested in isolation; integration testing, which verifies the interaction between modules; system testing, evaluating the entire system as a whole; and acceptance testing, confirming that the software meets user requirements. The notes also discuss regression testing to ensure that new code doesn’t introduce defects into existing functionality. Furthermore, the importance of test-driven development (TDD), where tests are written before the code, is highlighted. Different testing levels are described, along with their respective purposes and techniques. The notes may include examples and case studies to illustrate the application of these methodologies in real-world scenarios. A comprehensive understanding of software testing methodologies is essential for producing reliable and high-quality software products. The notes aim to provide a clear and practical guide to these essential techniques.
Software Quality Attributes
Software quality attributes are crucial characteristics that determine the overall quality and success of a software system. These notes explore key attributes, including functionality, which refers to the software’s ability to perform its intended functions correctly and efficiently. Reliability encompasses the software’s consistency in delivering expected results without failures. Usability focuses on the ease with which users can interact with and understand the system. Efficiency addresses the resource consumption (time and memory) of the software. Maintainability examines the ease of modifying and updating the software over time. Portability describes the ability to adapt the software to different environments and platforms. Security ensures the protection of the software and its data from unauthorized access and threats. These notes provide detailed explanations of each attribute, along with practical examples and guidelines for achieving high quality in each area. They may also discuss the trade-offs that often exist between different quality attributes, and how to prioritize them based on project requirements. The importance of measuring and assessing these attributes throughout the software development lifecycle is emphasized, as it ensures continuous improvement and high-quality results. Achieving high software quality requires a comprehensive understanding of these attributes and their interdependencies.
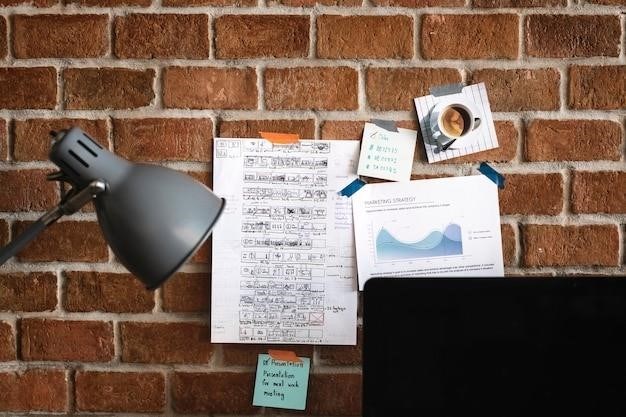
Software Project Management
Effective software project management is critical for successful software development. These notes cover planning, estimation, risk management, and other key aspects. They emphasize the importance of using established methodologies and tools for efficient project execution and delivery.
Software Project Planning and Estimation
Software project planning involves defining project goals, deliverables, timelines, and resources. Effective planning minimizes risks and maximizes the chances of on-time and within-budget project completion. Key aspects include defining clear project scope, creating detailed work breakdown structures (WBS), and establishing realistic schedules and milestones. This often involves utilizing project management tools and techniques such as Gantt charts and critical path analysis to visualize project dependencies and track progress. Accurate estimation of effort, cost, and resources is crucial for effective planning. Various estimation techniques, including expert judgment, analogous estimation, and parametric modeling, are employed to predict project resource needs. These estimations are often refined throughout the project lifecycle based on feedback and updated information. The notes emphasize the importance of iterative planning, allowing for adaptation as new information becomes available and to accommodate changing requirements.
Software Risk Management
Effective software risk management is crucial for successful software projects. Risks can stem from various sources, including technical challenges, changing requirements, resource constraints, and communication breakdowns. A systematic approach to risk management involves identifying potential risks, analyzing their likelihood and impact, and developing mitigation strategies. Risk identification techniques include brainstorming, checklists, and SWOT analysis. Qualitative risk analysis involves assessing the likelihood and impact of each identified risk, often using probability and severity matrices. Quantitative risk analysis uses numerical data to estimate the potential financial impact of risks. Mitigation strategies aim to reduce the likelihood or impact of risks, and may involve technical solutions, process improvements, or contingency planning. The notes also highlight the importance of risk monitoring and control throughout the project lifecycle. Regular risk reviews help track the status of identified risks and adjust mitigation strategies as needed. Proactive risk management minimizes disruptions and increases the probability of project success.